How to Create a Next.js App
Getting Started with Next.js: A Beginner’s Guide
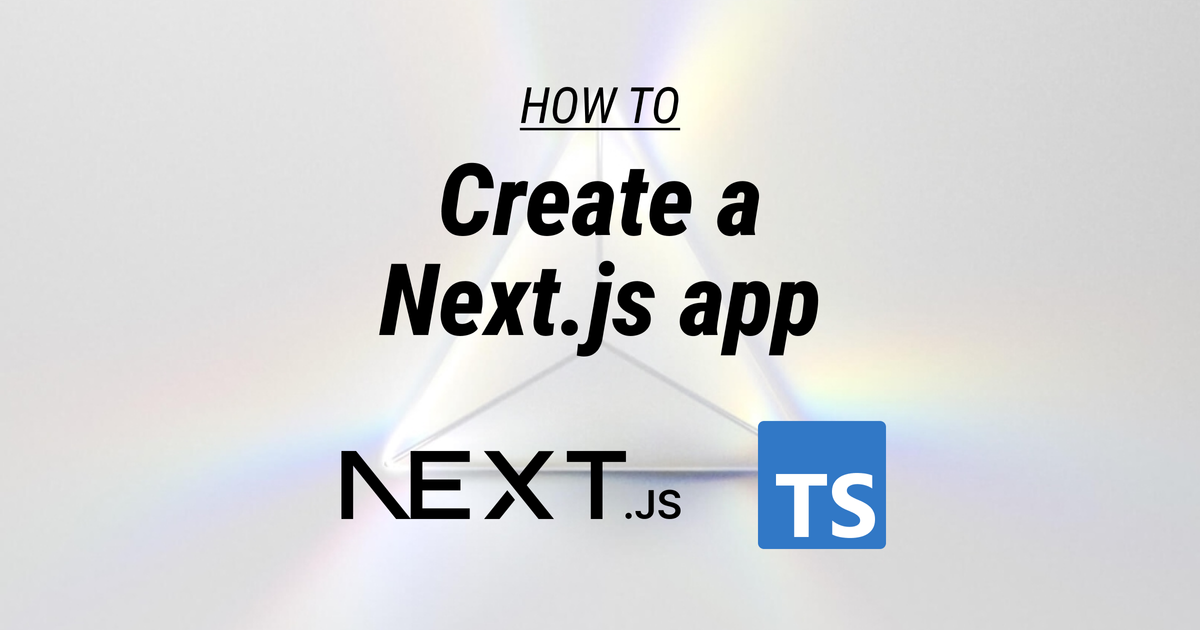
This post is part of my Next.js and TypeScript series — feel free to check out my other posts if this is something that interests you!
Next.js is a popular framework for building React applications. It makes it easier to create server-rendered React apps with benefits like automatic code splitting, and improved performance. In this tutorial, I’ll guide you through the process of setting up your first Next.js app. This tutorial is aimed at complete beginners, so don’t worry if you’ve never used Next.js or React before.
Prerequisites
- Node.js 18.17.0 or later
- A computer, running macOS, Windows, or Linux. You got one of those, right?
Automatic Installation
Alright, so the easiest way to set up a Next.js application is to use create-next-app
. This tool automates the setup process for you, but comes with certain defaults which might not be everyone’s cup of tea. Here's how to get started:
- Open your terminal.
- Run the following command:
npx create-next-app@latest
- During the installation, you’ll be prompted with a series of questions. Here’s a brief overview:
Project name: Choose a name for your project.
TypeScript: Decide whether you want to use TypeScript. That’s a yes from me!
ESLint: Choose whether you want to use ESLint for code quality. “Y” again.
Tailwind CSS: Decide if you want to use Tailwind CSS for styling. I personally prefer MUI for its ready-to-go components, but each to their own!src/
directory: Opt for asrc/
directory to organize your code. This kinda feels unnecessary to me.
App Router: You can choose to either use the new App Router method or the old Pages way of doing things. New applications should generally use App Router, so that’s what well cover in this guide.
Import alias: Configure the default import alias (e.g.,@/*
). This is again a bit more advanced for cleaner relative imports and monorepo packages. - Hit enter, and boom! Your Next.js project folder is ready.
After installation, you’ll get a bunch of files and folders. These are basically configurations and starter code to get your Next.js app up and running quickly. From here you can basically skip to the “Run the Development Server” section
Manual Installation
If you want to roll your sleeves up and do it manually, no worries. Create a new folder on your system, navigate into it, and open your terminal and run:
npm install next@latest react@latest react-dom@latest
Then, edit your package.json
file to include these scripts:
{
"scripts": {
"dev": "next dev",
"build": "next build",
"start": "next start",
"lint": "next lint"
}
}
These scripts will help you manage different stages of development:
- dev: Run
next dev
to start Next.js in development mode. - build: Use
next build
to build the application for production. - start: Launch a Next.js production server with
next start
. - lint: Set up Next.js’ built-in ESLint configuration using
next lint
.
Folder Structure
Next.js uses file-system routing, which means your application’s routes are determined by how you structure your files.
The app
Directory
The app
folder is used by the Next.js App Router to serve your pages. This supports shared layouts, nested routing, loading states, error handling, and more. To create a new page at the root of your application:
- Create the
app/
folder. - Inside the
app/
folder, add alayout.tsx
and apage.tsx
file. These files will be rendered when a user visits the root of your application (/
). - In
app/layout.tsx
, create the root layout:
export default function RootLayout({ children }: { children: React.ReactNode }) {
return (
<html lang="en">
<body>{children}</body>
</html>
)
}
- Finally, create the home page in
app/page.tsx
with some initial content.
export default function Page() {
return <h1>Welcome to Next.js!</h1>
}
The public
Folder
The public
folder exists for storing static assets like images, fonts, etc. Files inside the public
directory can be referenced by your code from the base URL (/
).
For example, if you were to put a file named avatar.png
in your public
folder, you would be able to reference it in your page app like so:
import Image from 'next/image'
export default function Page() {
return (
<div>
<h1>Welcome to Next.js!</h1>
<Image src="/avatar.png" alt="Avatar" width="64" height="64" />
</div>
)
}
Run the Development Server
Once your application is set up, all that’s left is to run the Next.js development server and have a look at your baby.
- Run the following command in your terminal:
npm run dev
- Open your web browser and go to http://localhost:3000 to view your application.
- Edit
app/layout.tsx
orapp/page.tsx
with some changes, and save the file. - You should now see the updated result in your browser!
What’s next?
You can now start building your Next.js application. Here are some tips to get you started:
- To add more pages, simply create a new folder inside the
app/
directory, name it something likeabout/
, and add apage.tsx
file inside it. Next.js will work its magic and you’ll be able to view it at http://localhost:3000/about. - You can create components right in the
app/{page}/
folder - no need to create a dedicatedcomponents/
folder if your application is not at that scale yet, although you totally could! Just make sure not to name any of thempage.tsx
orroute.ts
and you’ll be fine.
Wrapping Up
And there you have it! This was a very short intro in creating your very own Next.js app, and getting it up and running. In the next few guides in this series, we’ll dig deeper into adding more pages and styles, integrating with Tailwind and MUI, and dockerizing your Next.js application.
Until next time — happy coding!